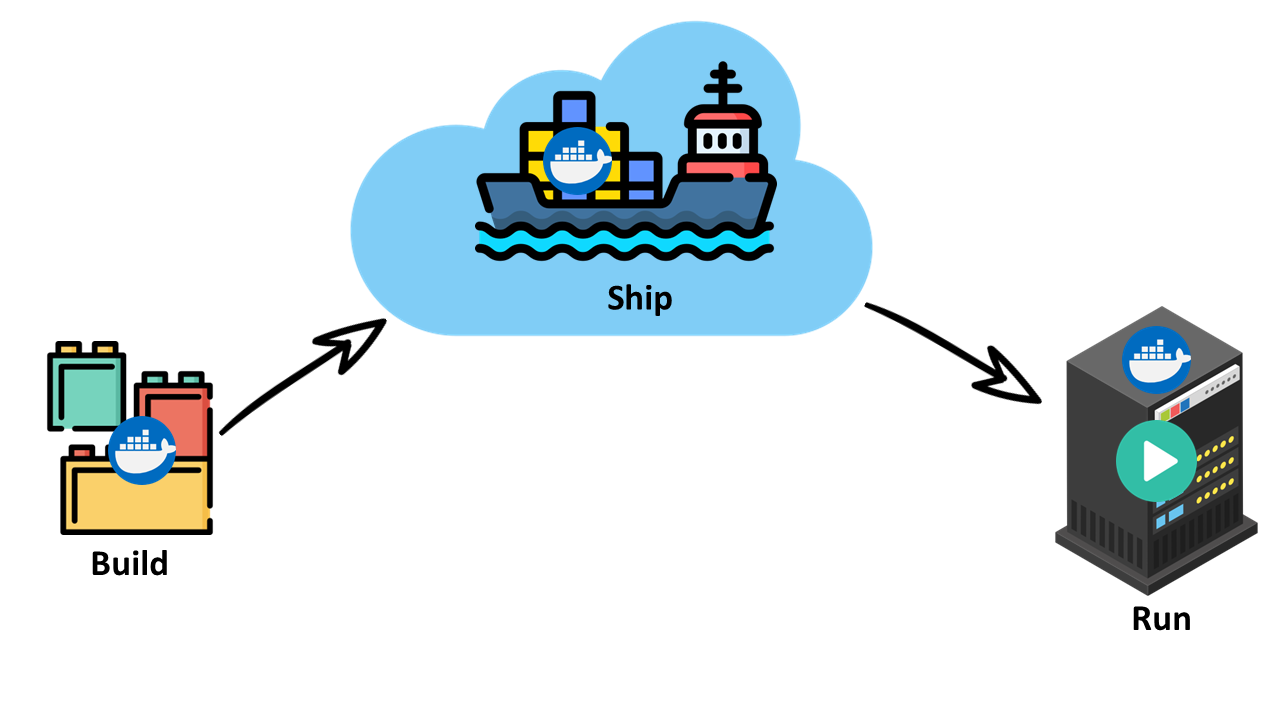
Introduction
Docker, an open-source platform, revolutionizes software development and deployment processes by automating the deployment of applications inside lightweight containers. This article will provide an in-depth look at Docker's built, ship, and run cycle, focusing on the container image, Dockerfile, image layers, Docker registry, universal app distribution, and finally, the Docker container.
Build, Ship and Run Cycle
The Docker build, ship, and run cycle is the backbone of Docker's utility in streamlining application deployment. The build phase involves creating a Dockerfile that defines a Docker image, which encapsulates the application along with its dependencies in a self-sufficient container. This container is then built into an image using the docker build command. This image is identified by an SHA hash that represents the unique combination of its layers. The ship phase involves storing the built image in a Docker Registry, a server-side application that enables global distribution of Docker images. This is achieved through the docker push command which uploads the image to the registry. The image can then be universally accessed and pulled onto any Docker-enabled machine using the docker pull command, ensuring consistent application operation across varied environments. The run phase is the final part of the cycle where the pulled Docker image is run on the host machine, creating a Docker container. This container provides an identical runtime environment, regardless of the host, to execute the application as defined, ensuring operational uniformity and reproducibility.
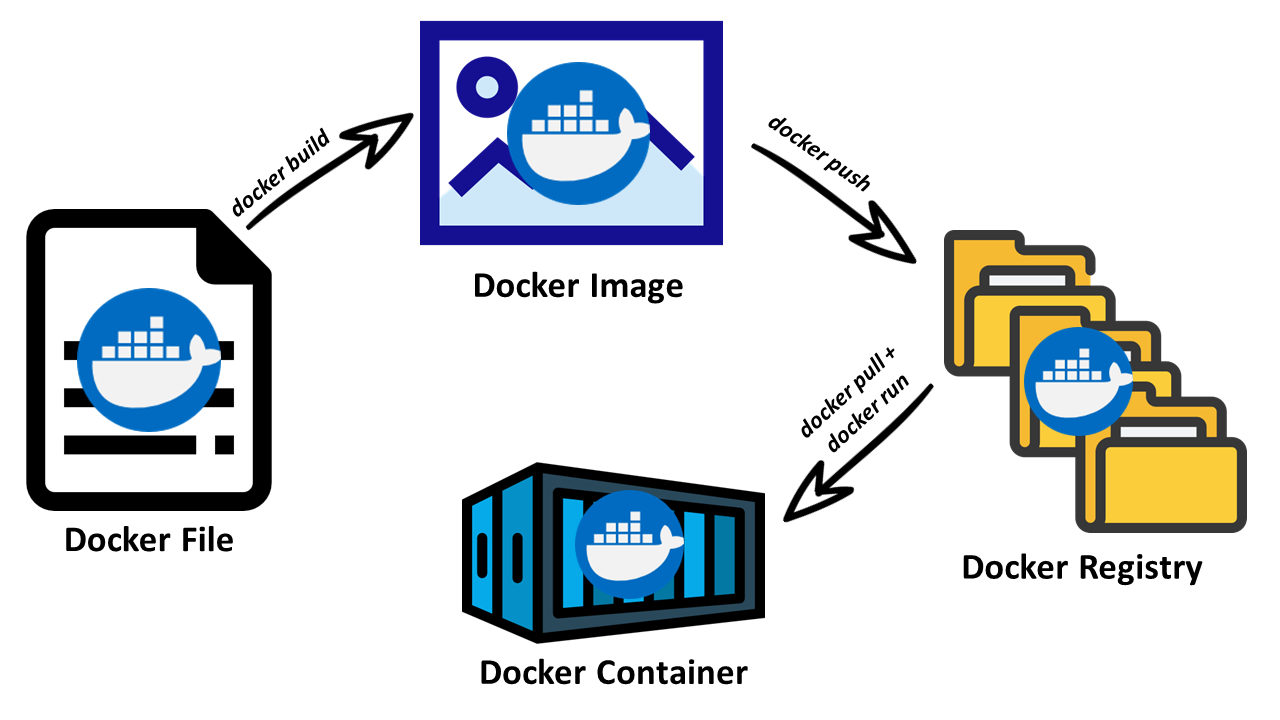
Dockerfile and Image Layers
A Dockerfile is a text document that contains all the commands a user could call on the command line to assemble an image. When Docker builds an image from a Dockerfile, it does so in layers. Each Dockerfile instruction creates a new layer in the image, with changes stored in the layer where they were made.
Here's a simple example of a Dockerfile for a Python app:
FROM python:3.8
WORKDIR /app
RUN pip install requests
COPY . .
CMD ["python", "app.py"]
Here's what each line does:
-
FROM python:3.8
: This line specifies the base image that will be used to run your application. In this case, it's using an image that has Python 3.8 installed. TheFROM
instruction initializes a new build stage and sets the base image for subsequent instructions. -
WORKDIR /app
: TheWORKDIR
instruction sets the working directory within the Docker container for any subsequent instructions. Here, it's setting the working directory to/app
. If the directory does not exist, Docker will create it. -
RUN pip install requests
: ThisRUN
instruction will execute the commandpip install requests
during the build of the Docker image. It's used to install the "requests" Python library in the image, which is a common library used for making HTTP requests in Python. -
COPY . .
: TheCOPY
instruction copies new files or directories from<src>
and adds them to the filesystem of the container at the path<dest>
. Here it's copying everything from the current directory (first.
) on the host to the current directory (second.
) in the container. Because theWORKDIR
was set to/app
, it means it'll copy the files into/app
inside the container. -
CMD ["python", "app.py"]
: TheCMD
instruction provides defaults for an executing container. This line is saying when a container is started from the image, by default it should run the commandpython app.py
. In other words, it will start your Python application by running theapp.py
file. Note that if you run the container and provide arguments (for example,docker run <image> <command>
), it will override the defaultCMD
.
This Dockerfile is a typical example for a simple Python application, specifying the environment, dependencies, and how to run the application.
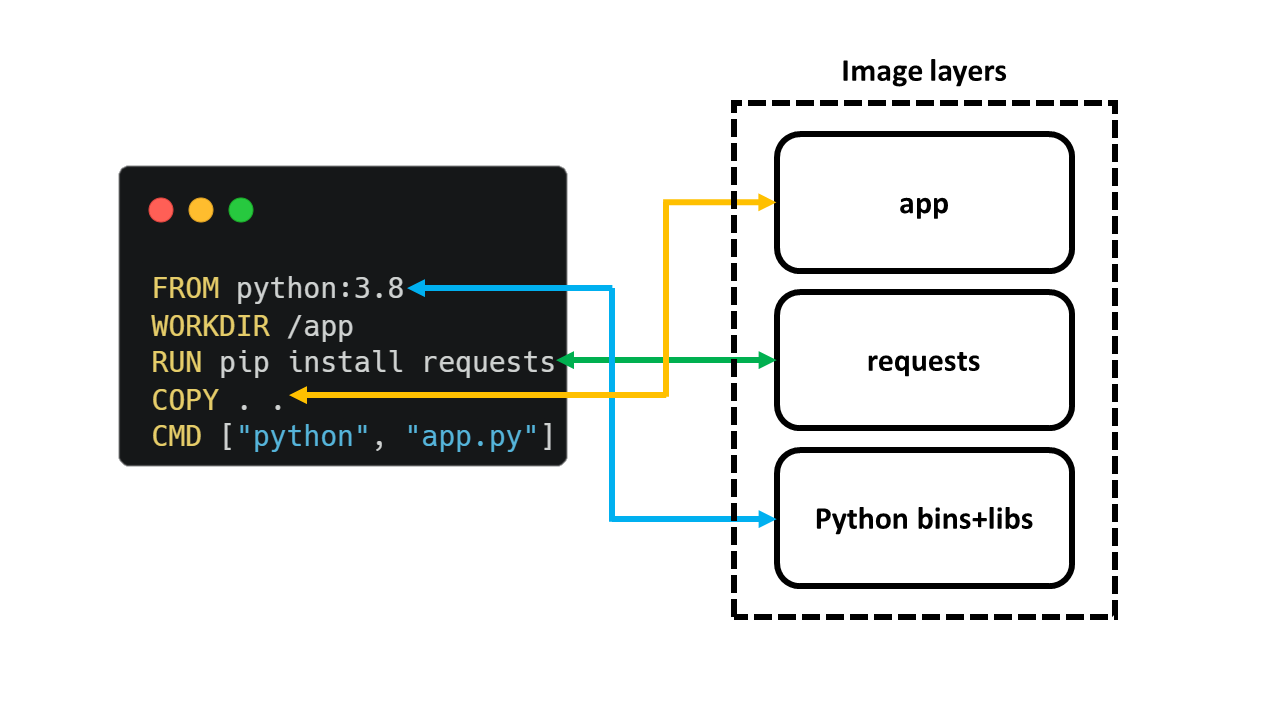
Built Image and Image SHA Hash
Once we've written the Dockerfile, we can build our image using the docker build
command:
docker build -t my-python-app .
This command tells Docker to build an image using the Dockerfile in the current directory (.
), and tag (-t
) the image as "my-python-app".
The output of this process is an image, identified by a SHA hash, which represents the unique identifier of all the layers in the image combined.
Container Images
A Docker container image is a lightweight, standalone, executable package that includes everything needed to run a piece of software, including the code, a runtime, libraries, environment variables, and config files. The image provides a consistent and reproducible environment, ensuring the application runs the same, regardless of where it is deployed.
Docker Registry
A Docker Registry is a server-side application that stores Docker images. Docker Hub and Google Container Registry are popular public registries, but you can also host your private registry.
Universal App Distribution
With the Docker image built, you can distribute it universally. It means anyone with Docker installed can pull and run your image, irrespective of the operating system or the environment settings. The command docker push
allows you to push your image to a registry:
docker push my-python-app
Docker Pull
On any other machine with Docker installed, you can pull the identical copy of the built image using the docker pull
command:
docker pull my-python-app
Docker Container and Identical Runtime Environments
A Docker container is a runtime instance of a Docker image. Containers encapsulate the application code and all its dependencies, ensuring it runs uniformly across different computing environments.
To run the image on Windows, you would use this command:
docker run -p 4000:80 my-python-app
This command tells Docker to run our image and map port 4000 on the host to port 80 on the Docker container.
Conclusion
Docker's built, ship, and run cycle is a powerful concept in the software development and deployment process. It simplifies the development process by creating a consistent environment through Dockerfiles and container images. The Docker Registry further enhances this process, providing a platform for universal app distribution. As a result, Docker has become an integral part of modern DevOps practices, enabling more efficient and reliable software development workflows.